Hi there!
Welcome to the first episode of Programming Fundamentals : Data Structures blog series. In this series, I will be covering a surface introduction to the data, data structures and types. In this first episode, I will briefly discuss data, data types and data structures.
I have divided the contents into different topics to make it easier to read and understand. For you to continue with this series, you must have a basic understanding of programming terminologies such as variables, loops, conditional statements etc. So, without further ado, let's dive straight into the topics.
So, what is data?
In this context, data is information that is stored or processed by a computer. It is simply numbers, letters etc. Simple examples of data would be your name, address, birthday etc. In a computer program, they are stored in different formats to organize and access. In depth, they are just binary sequences of 1s and 0s. For ease, different programming languages allow us to store data in different formats and they convert our data into computer understandable binary, under the hood. We don't need to bother about the abstract layers of functionalities so that we could focus on writing large programs.
Common types of data
The common types of data we interact with in programming are numbers, letters, true and false values etc. In programming languages, there are defined conventions on how these data are stored. These data are classified into different data types based on their values. A data type is basically an attribute of data that describes the values it can have and how that data can be used. In further sections, I will be discussing different data types.
Numeric data types
The data types that are used to store the numeric values are numeric data types. They can be Int, Double, Float, Short and Long. Most of them are available in all programming languages while some are language specific. The Int, Short and Long data types are used to store the whole numbers while Double and Float are used to store decimal numbers.
Why are there multiple data types to store just numbers?
The simple answer is because they differ in size of data they hold. Each of them has a defined length of number and decimal values they can store. Such as, in general, an Integer data type holds 32-bits of data while a Double can hold 64-bits of data.
// Some numeric data types in java
int a = -27;
short b = 100;
long c = 1927881L;
double d = 6535.34d;
float e = 8.454f;
Signed and Unsigned numeric data types
The data types I discussed in the previous section are signed numeric data types i.e. they can hold both negative and positive values. The unsigned data types are those which store only positive numeric values. The unsigned values need to be explicitly defined in the program and are supported in few programming languages like C, C++ etc. All numeric data types can be defined as unsigned. While defining the data types as unsigned, the range of values that data type supports are shifted.
For example: if a signed short data type holds a 16-bits value ranging from -32768 to 32767 then when it is defined unsigned, the supported value range becomes 0 to 65535 i.e. all positive values with 16-bits length being unchanged.
/* Signed and Unsigned ints in C */
int a = -27;
unsigned int b = 65;
Booleans
A Boolean data type stores one of two possible values which represents the true or false state of a logic. Booleans are simply either 0 or 1. A simple example would be of a normal electric switch, which can either be off or on at a time.
# Booleans in Python
switch_off = False
switch_on = True
Chars
A single symbol or letter is stored in a program using a character or char datatype. How many bits of data a char can hold, depends upon the programming language. For example: a char is 8-bits in C++ while it is 16-bits in Java, Kotlin, Swift etc.
// Char in Swift
var a: Character = "A"
Every data type discussed above are primitive data types. They have a defined size in memory which is independent of the value they hold. Let us see an example below to better understand it:
// Binary representation of int values
int a = 15 -> 00000000000000000000000000001111
int b = 2980 -> 00000000000000000000101110100100
In the above snippet, two integers were defined with value 15 and 2980. When the program stores these defined integers, it takes 32-bits space in the memory independent of the value stored in the variable.
Data Structures
Now we understand some primitive data types. So, why are data structures needed? Role of data structures comes into play if we want to store multiple ints or a series of chars in a variable. Simply, data structures are containers that hold several pieces of data in a single structure. Imagine it as different compartments in a departmental store with different items in each compartment based on the type of items. Similarly, data structures give us a way to organize, store and access the data efficiently.
To better understand it, let's consider a scenario where we want to keep a record of the number of pens each student has in a classroom.
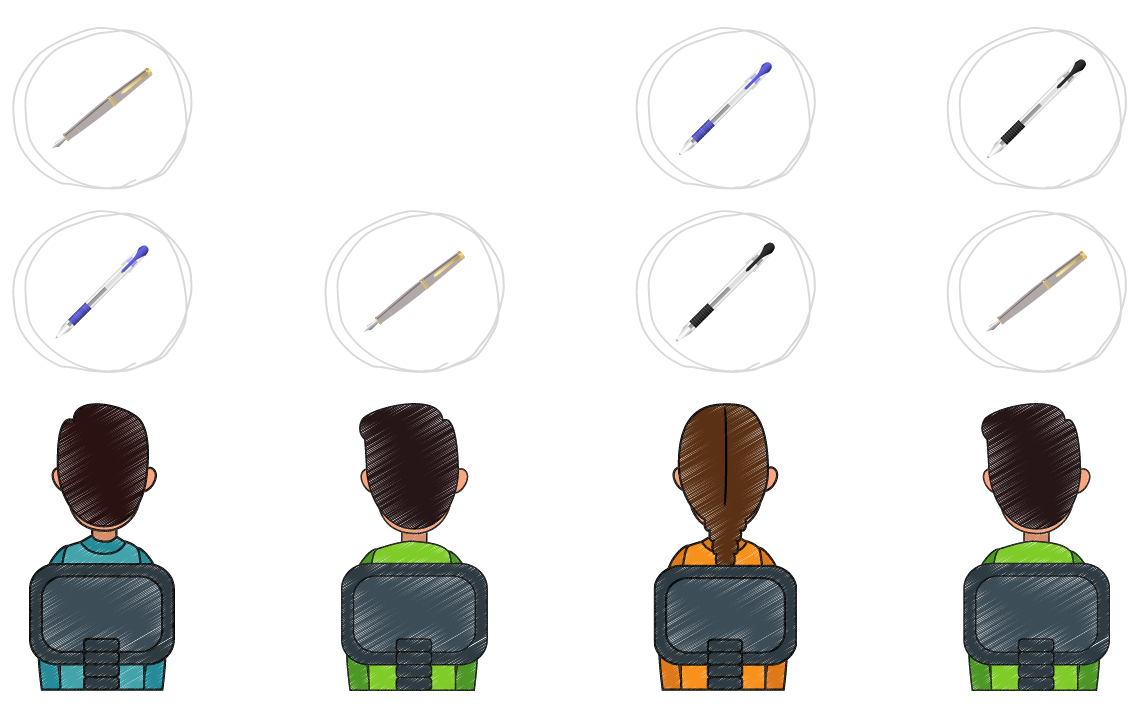
A simple way would be defining a separate variable for each student and recording the number.
int pensFirstStudent = 2;
int pensSecondStudent = 1;
int pensThirdStudent = 2;
.....
int pensNthStudent = 4;
Following this way, the record can be kept but this is not the right way to do it. It has some serious issues. The stored variables have no linkage at all. Without a link it is not very difficult to calculate the total number of pens, total number of a single type of pens, average number of pens etc. And it is very difficult to initialize a single variable for each student if the number of students is very large. So, the main aim of data structures is to organize and store the data correctly so that they can be accessed quickly and efficiently. A simple and good way to store this data would be:
pens = {2,1,2,3,2,1,0,0,2,0,1,2,...,4}
Let's not give this organization technique any name right now but it seems a simple and effective way to store. If someone wants to know how many pens does a second student have, one can simply query the pens variable in the second index. To calculate the total, the number in each index can be quickly summed and so on. We will discuss other techniques in upcoming posts of this series.
Strings
A string is a sequence of characters. It is a data type that's built out of another data type. So, it is not a primitive data type, instead it is an organized series of characters, and it is implemented using a data structure. The size of a string often depends on how we allocate the data or how much the size of each data piece takes.
// A String in Swift
let apple: String = "Apple"
// The word apple has five characters which are organized in a certain order to form a meaningful string Apple.
There are many other different data structures such as arrays, stacks, queues, graphs, trees etc. which will be covered in upcoming parts of this blog series. Subscribe to SubiDroid and stay tuned for updates.
If you have any queries, post them in the comment section.
Happy Learning! ❤️